Stop Unity from compiling every time you save a script in Visual Studio
It can quickly become a frustrating experience when one small change and save to a script causes your entire project to recompile in Unity when tabbing back and forth from Unity and Visual Studio (VS). This is especially frustrating if you tab back and forth frequently, only to be prompted with the script recompiling loading bar.
Googling this and looking for a solution on StackExchange, Unity discussion forum, among other places, the responses do not directly address the question. Some people have recommend disabling Unity Editor Auto Refresh in the General options in Unity 2021 or earlier, or Asset Pipeline in Unity 2022 or later. Unfortunately, this doesn’t respond to this specific issue. Another recommendation is to add Burst in Unity’s Package Manager – and while this improves and optimizes compilation, it does not stop every small save in VS from compiling. In fact, the solution is not found in Unity Editor, but rather, in VS which is refreshing Unity’s AssetDatabase through Tools for Unity every time a file is saved.
There’s a really simple solution in Visual Studio:
In Visual Studio 2019 or 2022, click Tools -> Options -> Tools for Unity -> Refresh Unity’s AssetDatabase on save -> Set to False.
Then close and reopen Unity and Visual Studio for the changes to take place. That’s it. And when you do want to compile, simply press Ctrl + R within the Unity Editor.
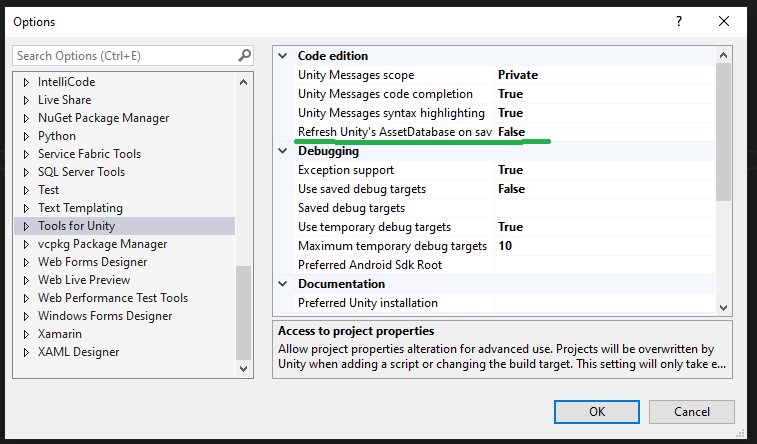